biotite.structure.io.pdbx.CIFFile¶
- class biotite.structure.io.pdbx.CIFFile(blocks=None)[source]¶
Bases:
_Component
,File
,MutableMapping
This class represents a CIF file.
The categories of the file can be accessed and modified like a dictionary. The values are
CIFBlock
objects.To parse or write a structure from/to a
CIFFile
object, use the high-levelget_structure()
orset_structure()
function respectively.Notes
The content of CIF files are lazily deserialized: When reading the file only the line positions of all blocks are indexed. The time consuming deserialization of a block/category is only performed when accessed. The deserialized
CIFBlock
/CIFCategory
objects are cached for subsequent accesses.Examples
Read a CIF file and access its content:
>>> import os.path >>> file = CIFFile.read(os.path.join(path_to_structures, "1l2y.cif")) >>> print(file["1L2Y"]["citation_author"]["name"].as_array()) ['Neidigh, J.W.' 'Fesinmeyer, R.M.' 'Andersen, N.H.'] >>> # Access the only block in the file >>> print(file.block["entity"]["pdbx_description"].as_item()) TC5b
Create a CIF file and write it to disk:
>>> category = CIFCategory( ... {"some_column": "some_value", "another_column": "another_value"} ... ) >>> block = CIFBlock({"some_category": category, "another_category": category}) >>> file = CIFFile({"some_block": block, "another_block": block}) >>> print(file.serialize()) data_some_block # _some_category.some_column some_value _some_category.another_column another_value # _another_category.some_column some_value _another_category.another_column another_value # data_another_block # _some_category.some_column some_value _some_category.another_column another_value # _another_category.some_column some_value _another_category.another_column another_value # >>> file.write(os.path.join(path_to_directory, "some_file.cif"))
- Attributes
- blockCIFBlock
The sole block of the file. If the file contains multiple blocks, an exception is raised.
- clear() None. Remove all items from D. ¶
- copy()¶
Create a deep copy of this object.
- Returns
- copy
A copy of this object.
- static deserialize(text)¶
Create this component by deserializing the given content.
- Parameters
- contentstr or dict
The content to be deserialized. The type of this parameter depends on the file format. In case of CIF files, this is the text of the lines that represent this component. In case of BinaryCIF files, this is a dictionary parsed from the MessagePack data.
- get(k[, d]) D[k] if k in D, else d. d defaults to None. ¶
- items() a set-like object providing a view on D's items ¶
- keys() a set-like object providing a view on D's keys ¶
- pop(k[, d]) v, remove specified key and return the corresponding value. ¶
If key is not found, d is returned if given, otherwise KeyError is raised.
- popitem() (k, v), remove and return some (key, value) pair ¶
as a 2-tuple; but raise KeyError if D is empty.
- classmethod read(file)¶
Read a CIF file.
- Parameters
- filefile-like object or str
The file to be read. Alternatively a file path can be supplied.
- Returns
- file_objectCIFFile
The parsed file.
- serialize()¶
Convert this component into a Python object that can be written to a file.
- Returns
- contentstr or dict
The content to be serialized. The type of this return value depends on the file format. In case of CIF files, this is the text of the lines that represent this component. In case of BinaryCIF files, this is a dictionary that can be encoded into MessagePack.
- setdefault(k[, d]) D.get(k,d), also set D[k]=d if k not in D ¶
- static subcomponent_class()¶
Get the class of the components that are stored in this component.
- Returns
- subcomponent_classtype
The class of the subcomponent. If this component already represents the lowest level, i.e. it does not contain subcomponents,
None
is returned.
- static supercomponent_class()¶
Get the class of the component that contains this component.
- Returns
- supercomponent_classtype
The class of the supercomponent. If this component present already the highest level, i.e. it is not contained in another component,
None
is returned.
- update([E, ]**F) None. Update D from mapping/iterable E and F. ¶
If E present and has a .keys() method, does: for k in E: D[k] = E[k] If E present and lacks .keys() method, does: for (k, v) in E: D[k] = v In either case, this is followed by: for k, v in F.items(): D[k] = v
- values() an object providing a view on D's values ¶
- write(file)¶
Write the contents of this object into a CIF file.
- Parameters
- filefile-like object or str
The file to be written to. Alternatively a file path can be supplied.
Gallery¶
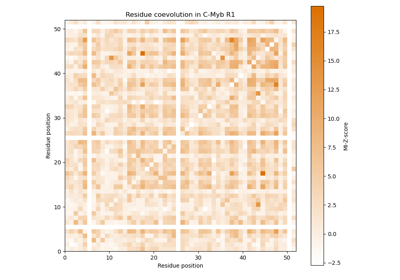
Mutual information as measure for coevolution of residues